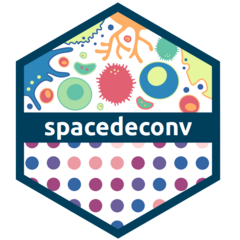
Clustering
spacedeconv_clustering.Rmd
To gain more insights into a tissues composition the clustering function can be applied. It is possible to cluster by expression values, deconvolution results or pathway and transcription factor activities.
library(spacedeconv)
library(SpatialExperiment)
data("single_cell_data_3")
data("spatial_data_3")
single_cell_data_3 <- spacedeconv::preprocess(single_cell_data_3)
spatial_data_3 <- spacedeconv::preprocess(spatial_data_3)
single_cell_data_3 <- spacedeconv::normalize(single_cell_data_3, method = "cpm")
spatial_data_3 <- spacedeconv::normalize(spatial_data_3, method = "cpm")
signature <- spacedeconv::build_model(
single_cell_obj = single_cell_data_3,
cell_type_col = "celltype_major",
method = "dwls", verbose = T, dwls_method = "mast_optimized", ncores = 10
)
deconv <- spacedeconv::deconvolute(
spatial_obj = spatial_data_3,
single_cell_obj = single_cell_data_3,
cell_type_col = "celltype_major",
method = "dwls",
signature = signature,
assay_sp = "cpm"
)
First we show how to cluster deconvolution data. Set the data parameter to “deconvolution” and provide the deconvolution tool you used. You can further set the following parameters:
- nclusters: Number of clusters you want, can be a range
- spmethod: should be the deconvolution tool used, or progeny/dorothea when clustering decoupleR results
- method: kmeans or hclust
- dist_method: for hclust, which distance method to use (“correlation”, “euclidean”, “maximum”, “manhattan”, “canberra”, “binary”, “minkowski”)
- hclust_method: for hclust, agglomeration method to us (“complete”, “ward.D”, “ward.D2”, “single”, “average”, “mcquitty”, “median”, “centroid”)
This function applies the Seurat clustering approach in the background. Set data to “expression”, this will use “counts” values for clustering. You can further set the following parameters:
- clusres: Cluster resolution, check the Seurat Vignette for details.
- pca_dim: Number of PCA dimensions to use
cluster <- spacedeconv::cluster(deconv, data = "expression", clusres = 0.5)
cluster <- readRDS(system.file("extdata", "cluster.rds", package = "spacedeconv"))
plot_celltype(cluster, "cluster", density = F) # plot the clustering stored in this object
With an available clustering you can exract the top features for each cluster. Here we extract the top features for each cluster based on expression, but we want the top features from the deconvolution results from this area. See the associated clusters in the plot above.
get_cluster_features(cluster, clusterid = "cluster_expression_0.5", spmethod = "dwls")
#> $`0`
#> dwls_Cancer.Epithelial dwls_PVL dwls_B.cells
#> 1.48622765 -0.09684142 -0.20176392
#>
#> $`1`
#> dwls_T.cells dwls_Endothelial dwls_PVL
#> 0.7706055 0.6349723 0.5992391
#>
#> $`2`
#> dwls_CAFs dwls_Normal.Epithelial dwls_Endothelial
#> 0.8553145 0.4136625 0.3882446
#>
#> $`3`
#> dwls_Myeloid dwls_Plasmablasts dwls_CAFs
#> 1.4167673 1.0846283 0.6236146
#>
#> $`4`
#> dwls_Normal.Epithelial dwls_PVL dwls_Endothelial
#> 2.02138850 0.08999605 -0.05634981
#>
#> $`5`
#> dwls_Cancer.Epithelial dwls_Myeloid dwls_T.cells
#> 0.83406399 0.72173387 0.06103056